First thing to note is to delete a Dynamics AX In-Bound port, you must ensure that the port is Deactive. In case you try to delete an Active port, you will get an error message similar to below.
The port 'InBound port name' must be undeployed before it can be deleted.
Just click button Deactivate after selecting the port you want to delete.
Path: System administration > Setup > Services and Application Integration Framework > Inbound ports
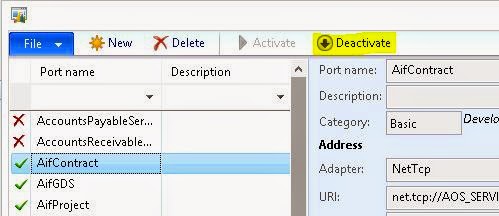
Another issue which you might have encountered while trying to delete an InBound port is the error message – “Port cannot be deleted while dependent Gateway queue exist. Delete dependent Gateway queue and try again.”
This happens when you have dependent records in Queue manager. The solution is to go to Queue manager and delete all the records which are using the port you want to delete.
Path: System administration > Periodic > Services and Application Integration Framework > Queue manager
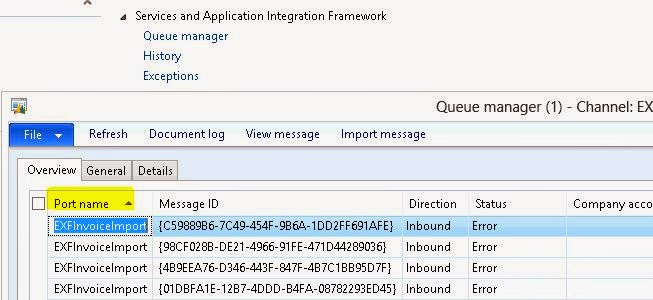
The port 'InBound port name' must be undeployed before it can be deleted.
Just click button Deactivate after selecting the port you want to delete.
Path: System administration > Setup > Services and Application Integration Framework > Inbound ports
Another issue which you might have encountered while trying to delete an InBound port is the error message – “Port cannot be deleted while dependent Gateway queue exist. Delete dependent Gateway queue and try again.”
This happens when you have dependent records in Queue manager. The solution is to go to Queue manager and delete all the records which are using the port you want to delete.
Path: System administration > Periodic > Services and Application Integration Framework > Queue manager